 |
Some browsers do not play video in WebGL with Unity 2020 and Unity VideoPlayer, see the chart below. In that case if your video is short, you could make a sprite sheet, or animated texture, see below.
Video Plugin, AV Pro You may want to use this $200 plugin because it can play many video codecs including HAP. Unity video player is limited.
Works with Mac, PC, WebGL, and all browsers. Do not use Unity 2020 yet.
In Unity Choose Window->Asset Store Type AVPro Video (Windows or Mac) in the search bar, Select the one that is $150 Pay and download
The AVProVideo folder and the Plugins folder need to be in your Unity project Assets folder. You can copy these between projects.
The StreamingAssets folder was created by AVPro, in your Assets folder. Put your movies there.
Add Media Player Object(s) to your Hierarchy tab Select GameObject->AVpro->Media Player for each video In the Inspector on the right: Click BROWSE to add your movie (located in your StreamingAssets folder) Select Playback Loop Under the Platform Specific button Select Windows or WebGL or Mac (For a Windows Player: Select Preferred Video API choose Direct Show)
Select your object Use Add Component->AVPro Video->Apply to Mesh in the Inspector Change Media to one of your Media Players Change Mesh to match the name of your object. (especially on Mac) If you like you can make a texture map of the first frame of your video and use it as the Default texture.
Delete any extra files in your StreamingAssets folder, they will get published.
Mac Bugs: Mac does not preview locally in browser, you must publish to a website. If you are having trouble with WebGL and video, test the project by publishing to the web. Local webGL publishes do not always work, especially on the Mac.
In addition check the permissions for the video file on the server.
Unity Video Player pretty disfunctional in 2019 Works with WebGL, on PC, works on Mac, does not work with Chrome at all. Use AVpro if you want video to work with webGL
MP4 h.264 or h.265 Video Texture map These formats might also work, depending on the codec: .mov .mpg .mpeg .mp4 .avi .asf Keep the pixel size small such as 426x240. Keep the bitrate low such as 300-700 Kbps. The framerate should not be over 30 fps. Keep the video duration short.
Make a folder in your Assets folder called StreamingAssets. Place a video file in the Assets folder of your Unity project.
Select an object in the Hierarchy that will play video. Select Add Component in the Inspector Choose Video->Video Player Change the Source to URL Hit Browse... to find your movie in your Assets/StreamingAssets folder. Turn on Loop if you prefer. If the video does not have have audio, turn off Audio Output Mode
Create a new material shader Assets->Create->Material Select Shader->Unlit/Texture (In the Inspector) (You might prefer the Standard Shader.) Drag the Material onto your object
Play the Game to see the texture
You can also attach a Video Player to your camera as a background.
When you publish save a copy of the StreamingAssets folder to the player folder, same level as Build, if you need to.
Fix BUG for VideoPlayer WebGL publish Make a script add to objects that have a video texture.
Select the your model
Use the Add Component button in the Inspector Scroll down to New script Type the name: fix_video_path_webgl Double click the new C# script in your Assets folder
Replace entire script with this script: using System.Collections; using System.Collections.Generic; using UnityEngine; using UnityEngine.Video;
public class fix_video_path_webgl : MonoBehaviour { public string URLvideo = "gulch.mp4";
// Start is called before the first frame update void Start() { GetComponent<VideoPlayer>().url = Application.streamingAssetsPath + "/" + URLvideo; } }
Change the video file name in the Inspector.
Add 3D sound to videoPlayer script By Alex Rickett UCLA Game Lab Unity does not provide 3D sound for the videoPlayer.
using System; using System.Collections; using System.Collections.Generic; using UnityEngine; using UnityEngine.Video;
public class WebGLVideoPseudo3DSound : MonoBehaviour {
public float minVolumeDistance = 10; public float maxVolumeDistance = 1; public float minVolume = 0; public float maxVolume = 1;
VideoPlayer _vp; private void Start() { _vp = this.GetComponent<VideoPlayer>(); minVolumeDistance *= minVolumeDistance; maxVolumeDistance *= maxVolumeDistance; if (!_vp.canSetDirectAudioVolume) { Debug.LogError("can't change volume! canSetDirectAudioVolume == false"); Destroy(this); } }
void Update() { float distance = (Camera.main.transform.position - this.transform.position).sqrMagnitude; float normDistance = Mathf.InverseLerp(minVolumeDistance, maxVolumeDistance, distance); float volume = Mathf.Lerp(minVolume, maxVolume, normDistance); _vp.SetDirectAudioVolume(0, volume); } }
FAKE texture map TV Animate texture offset to make a fake TV
Make an object with very small UV coordinates.
Create a new material, Assets->Create Material Change the Albedo color to black Select Emission Drag a map onto the Emission Color Map
Assign the Material to your model
Select the your model Use the Add Component button in the Inspector Scroll down to New script Type the name: fake_TV Double click the new C# script in your Assets folder
Replace entire script with this script: using System.Collections; using System.Collections.Generic; using UnityEngine;
public class fake_TV : MonoBehaviour { public float scrollSpeed = 0.2F; private Renderer rend;
void Start() { rend = GetComponent<Renderer>(); } void Update() { float offset = Time.time * scrollSpeed; rend.material.SetTextureOffset("_MainTex", new Vector2(offset, 0)); } }
Sprite Sheet for Short Low Resolution Video or Animation You will use an image sequence to make a single image that contains all the frames. A script will play the sheet as an animation texture map.
Use After Effects or any program to generate a short animation sequence.
Download and install GlueIT to create a sprite sheet.
Run GlueIT: Select Add, choose all your files. (png works, the images need to be the same size.) Input the number of columns Save your file into your Assets folder. Give it an informative name such as sheet_11columns_20rows_220_frames.png
How to calculate columns and rows: Unity has some texture limits. The default is 2048x2048. Keeping this number low and exponential will help performance. My sequence has 220 180x100 images 2048 / 180 pixels = 11.37, so I could use 11 columns. 2048 / 100 pixels = 20.48, so I could use up to 20 rows 11 columns x 20 rows = 220 frames My sheet will be 1980x2000 pixels
Create a Sprite Sheet Shader In Unity: Select Assets->Create->Shader->Unlit Shader A shader is code to generate an image. Name the shader test_Shader Double Click the shader in your Assets to edit the script.
Replace all the code with this script: Shader "Custom/test_Shader" { Properties { [Header(General)] _Color("Color", Color) = (1,1,1,1) _Glossiness("Smoothness", Range(0,1)) = 1 _Metallic("Metallic", Range(0,1)) = 1 [Header(Textures)] _MainTex ("Color Spritesheet", 2D) = "white" {} _NormalTex ("Normals Spritesheet", 2D) = "white" {}
[Header(Spritesheet)] _Columns("Columns (int)", int) = 3 _Rows("Rows (int)", int) = 3 _FrameNumber ("Frame Number (int)", int) = 0 _TotalFrames ("Total Number of Frames (int)", int) = 9 _Cutoff ("Alpha Cutoff", Range(0,1)) = 1 _AnimationSpeed ("Animation Speed", float) = 0 } SubShader { Tags { "RenderType"="Opaque" "DisableBatching"="True"} LOD 200 Cull Off
CGPROGRAM #pragma surface surf Standard fullforwardshadows alphatest:_Cutoff addshadow #pragma target 3.0
sampler2D _MainTex; sampler2D _NormalTex;
struct Input { float2 uv_MainTex; float4 color : COLOR; };
float4 _Color; half _Glossiness; half _Metallic; int _Columns; int _Rows; int _FrameNumber; int _TotalFrames;
float _AnimationSpeed;
void surf (Input IN, inout SurfaceOutputStandard o) {
_FrameNumber += frac(_Time[0] * _AnimationSpeed) * _TotalFrames;
float frame = clamp(_FrameNumber, 0, _TotalFrames);
float2 offPerFrame = float2((1 / (float)_Columns), (1 / (float)_Rows));
float2 spriteSize = IN.uv_MainTex; spriteSize.x = (spriteSize.x / _Columns); spriteSize.y = (spriteSize.y / _Rows);
float2 currentSprite = float2(0, 1 - offPerFrame.y); currentSprite.x += frame * offPerFrame.x; float rowIndex; float mod = modf(frame / (float)_Columns, rowIndex); currentSprite.y -= rowIndex * offPerFrame.y; currentSprite.x -= rowIndex * _Columns * offPerFrame.x; float2 spriteUV = (spriteSize + currentSprite); //* _FrameScale
fixed4 c = tex2D(_MainTex, spriteUV) * _Color;
o.Normal = UnpackNormal(tex2D(_NormalTex, spriteUV)); o.Metallic = _Metallic; o.Smoothness = _Glossiness; //change Albedo to Emission for backlit o.Albedo = c.rgb; o.Alpha = c.a; } ENDCG } FallBack "Transparent/Cutout/Diffuse" }
Create a Material Select Assets->Create->Material Name the material In the Inspector change the Shader option to your test_Shader, it is listed under Custom Change the number of Columns and Rows to match you sheet. Leave Frame Number 0 Input the Total Number of Frames Input the Animation Speed 1 Drag the sheet png onto Color Texture
Drag the material onto your model.
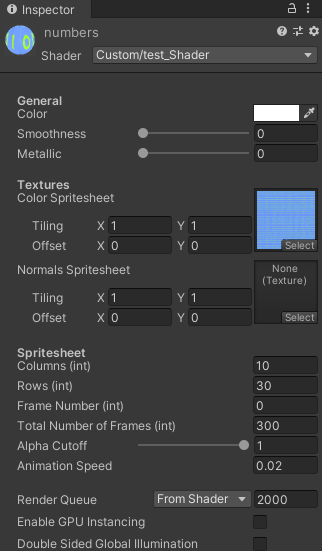
WebGL Video
|
|
|
12/4/2020
|
Unity VideoPlayer
|
local publish
|
web publish
|
|
Mac 2019.4.15
|
no
|
edge yes, safari yes, pc firefox yes*, mac firefox no, mac chrome no, pc chrome no
|
|
Mac 2020.1.16
|
no
|
edge yes, safari no, pc firefox no, mac firefox no, mac chrome no, pc chrome no
|
|
PC 2019.4.15
|
yes
|
edge yes, safari yes, pc firefox yes*, mac firefox no, mac chrome no, pc chrome no
|
|
PC 2020.1.15
|
yes
|
edge yes, safari no, pc firefox no, mac firefox no, mac chrome no, pc chrome no
|
|
|
Unity VideoPlayer needs a URL redirect script.
|
|
|
|
|
|
AVPRO Video Plugin
|
local publish, Build & Run
|
web publish
|
AVPro version
|
Mac 2019.4.11
|
firefox/chrome**
|
edge yes, safari yes, pc firefox yes, mac firefox yes, mac chrome yes, mac pc yes
|
1.11.6 1.11.7
|
Mac 2019.4.12
|
firefox/chrome**
|
edge yes, safari yes, pc firefox yes, mac firefox yes, mac chrome yes, mac pc yes
|
1.11.6. 1.11.7
|
Mac 2019.4.15
|
firefox/chrome**
|
edge yes, safari yes, pc firefox yes, mac firefox yes, mac chrome yes, mac pc yes
|
1.11.6. 1.11.7
|
Mac 2020.4.16 ***perm.
|
firefox/chrome**
|
edge yes, safari no, pc firefox no, mac firefox no, mac chrome no audio, pc chrome no audio
|
1.11.6. 1.11.7
|
PC 2019.4.11
|
yes
|
edge yes, safari yes, pc firefox* yes, mac firefox yes, mac chrome yes, mac pc yes
|
1.11.6. 1.11.7
|
PC 2019.4.15
|
yes
|
edge yes, safari yes, pc firefox* yes, mac firefox yes, mac chrome yes, mac pc yes
|
1.11.6. 1.11.7
|
PC 2020.4.15
|
yes
|
edge yes, safari no, pc firefox no, mac firefox no, mac chrome yes, pc chrome yes
|
1.11.6. 1.11.7
|
|
**Mac set default browser to firefox or chrome, no audio for local publish. Safari does not display the video with a local publish
|
|
***Mac intermittent permissions issue, check the permissions of your videos on the server, all users should be set to read
|
|
|
|
|
|
|
|
*Firefox video loops with a black frame and has an error message, but otherwise works.
|
|
SteamVR general issues
|
web publish
|
|
|
Mac 2019.4.15
|
no all browsers and no video
|
|
|
Mac 2020.1.15
|
no all browsers, safari black, firefox black, chome no video
|
|
PC 2019.4.15
|
edge yes, safari yes, pc firefox yes*, mac firefox no, mac chrome no, pc chrome no
|
|
PC 2020.1.15
|
edge yes, safari no, pc firefox no, mac firefox no, mac chrome no, pc chrome no
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|
|